Introduction to Events and Listeners in Laravel
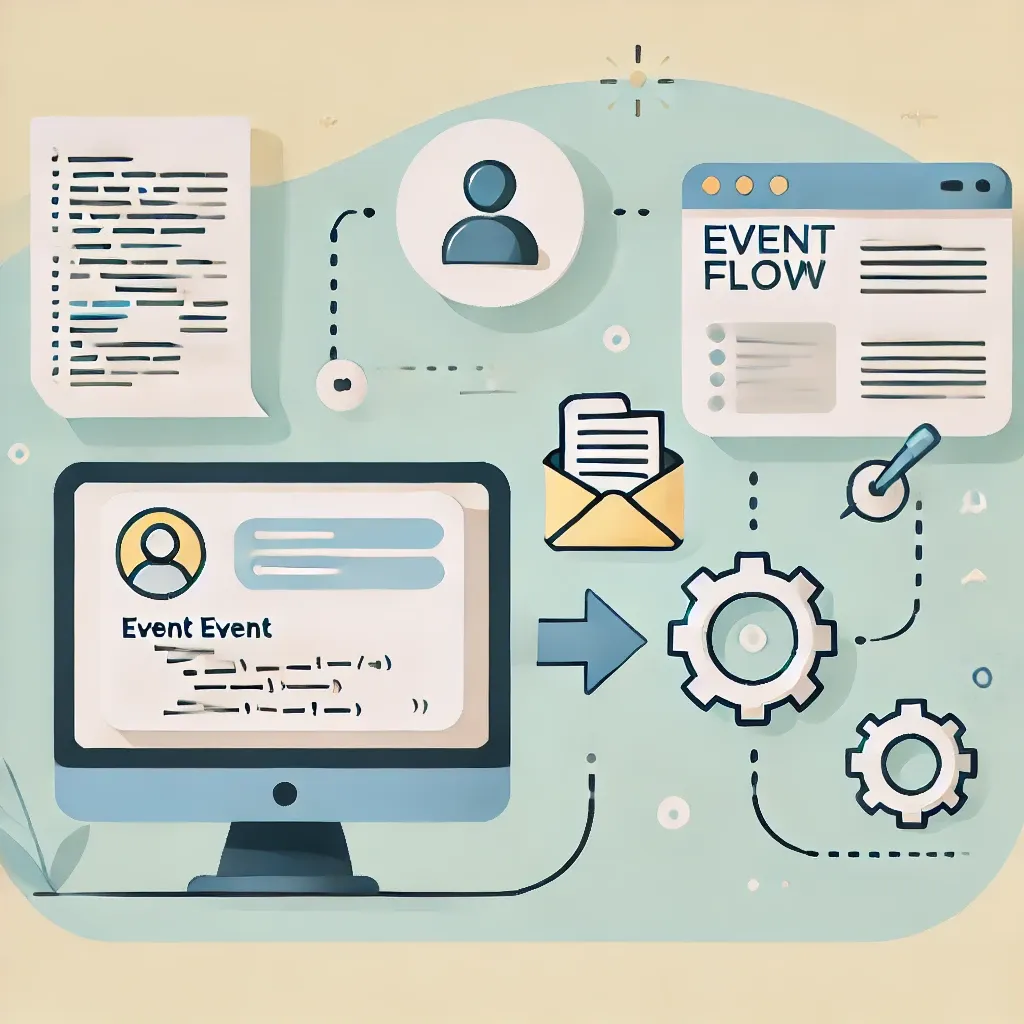
Events and listeners in Laravel are powerful tools that help you keep your code clean and organized by decoupling different parts of your application. They allow you to respond to specific actions, like user registration, without tightly coupling the business logic.
Let’s walk through creating a simple event and listener in Laravel.
Example: Logging a Message When a User Registers
We’ll create an event that is triggered when a user registers and a listener that logs a message.
Step 1: Generate the Event and Listener
Run the following Artisan commands to create an event and a listener:
php artisan make:event UserRegistered
php artisan make:listener LogUserRegistration --event=UserRegistered
These commands will generate two files:
- UserRegistered.php in the App\Events directory.
- LogUserRegistration.php in the App\Listeners directory.
Step 2: Define the Event
In UserRegistered.php, add the necessary properties and constructor to pass the user data:
namespace App\Events;
use App\Models\User;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class UserRegistered
{
use Dispatchable, SerializesModels;
public $user;
public function __construct(User $user)
{
$this->user = $user;
}
}
Step 3: Define the Listener
In LogUserRegistration.php, handle the event:
namespace App\Listeners;
use App\Events\UserRegistered;
use Illuminate\Support\Facades\Log;
class LogUserRegistration
{
public function handle(UserRegistered $event)
{
Log::info('User registered: ' . $event->user->email);
}
}
Step 4: Register the Event and Listener
Open App\Providers\EventServiceProvider.php and register the event and listener:
protected $listen = [
\App\Events\UserRegistered::class => [
\App\Listeners\LogUserRegistration::class,
],
];
Step 5: Trigger the Event
Trigger the UserRegistered event in your controller or service after a user registers:
use App\Events\UserRegistered;
use App\Models\User;
public function register(Request $request)
{
$user = User::create($request->only(['name', 'email', 'password']));
event(new UserRegistered($user));
return response()->json(['message' => 'User registered successfully']);
}
Step 6: Test It
- Register a user through your app or API.
- Check the Laravel logs in storage/logs/laravel.log.
- You should see a log entry like:
User registered:
[email protected]
Conclusion
Laravel’s events and listeners make it easy to decouple your application logic and respond to specific actions seamlessly. This simple example should give you a good starting point to implement events and listeners in your projects!